Understanding the Differences Between let, const, and var in JavaScript
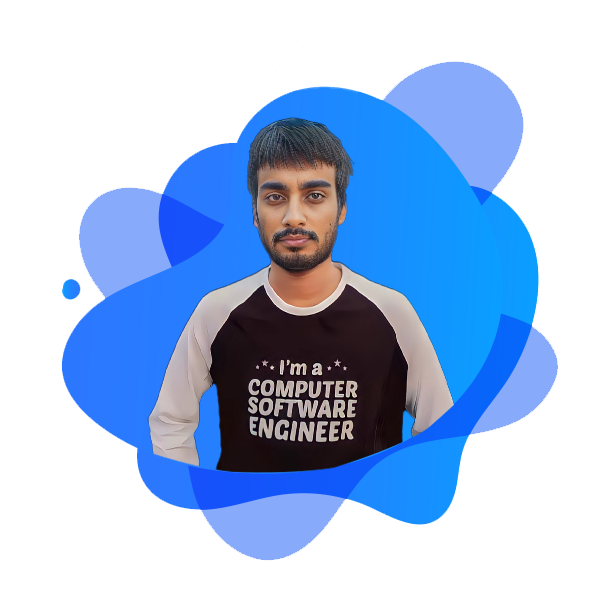
Hazrat Ali
Q1: What are let
, const
, and var
in JavaScript?
let
, const
, and var
are keywords used to declare variables in JavaScript.
-
var
: The oldest keyword, available since the beginning of JavaScript, but often avoided in modern code due to its quirks. -
let
: Introduced in ES6 (2015), it provides block scoping and better control over variables. -
const
: Also introduced in ES6, it’s used to declare variables that won’t be reassigned.
Q2: What is the scope of let
, const
, and var
?
Scope Comparison
-
var
:-
Function-scoped: The variable is accessible throughout the entire function it’s declared in.
-
If declared outside a function, it becomes globally scoped.
-
function testVar() {
if (true) {
var x = 10; // Function scoped
}
console.log(x); // Output: 10
}
-
let
andconst
:- Block-scoped: The variable is only accessible within the block
{}
where it’s declared.
- Block-scoped: The variable is only accessible within the block
function testLetConst() {
if (true) {
let y = 20;
const z = 30;
}
console.log(y); // ReferenceError
console.log(z); // ReferenceError
}
Q3: Can you redeclare variables with let
, const
, and var
?
-
var
:
Variables can be redeclared in the same scope without an error.var a = 10; var a = 20; // Allowed console.log(a); // Output: 20
-
let
andconst
:
Redeclaring variables in the same scope results in a syntax error.let b = 10; let b = 20; // SyntaxError: Identifier 'b' has already been declared
Q4: Are these variables hoisted?
All three are hoisted to the top of their scope, but their behavior differs:
-
var
:
Variables are hoisted and initialized asundefined
. This allows accessing them before their declaration.console.log(c); // Output: undefined var c = 10;
-
let
andconst
:
Variables are hoisted but remain in the Temporal Dead Zone (TDZ) until their declaration. Accessing them before the declaration results in aReferenceError
.console.log(d); // ReferenceError: Cannot access 'd' before initialization let d = 10;
Q5: Can you reassign values to these variables?
-
var
andlet
:
Both allow reassignment.var e = 10; e = 20; // Allowed console.log(e); // Output: 20 let f = 30; f = 40; // Allowed console.log(f); // Output: 40
-
const
:
Reassignment is not allowed. Once declared, aconst
variable’s value cannot be changed.const g = 50; g = 60; // TypeError: Assignment to constant variable
However, the contents of objects or arrays declared with
const
can still be modified.const obj = { key: 'value' }; obj.key = 'newValue'; // Allowed console.log(obj); // Output: { key: 'newValue' }
Q6: Do these variables attach to the global object?
-
var
:
Globally declaredvar
variables become properties of the global object (window
in browsers orglobal
in Node.js).var h = 10; console.log(window.h); // Output: 10
-
let
andconst
:
These variables do not attach to the global object.let i = 20; console.log(window.i); // Output: undefined
Q7: When should I use let
, const
, or var
?
-
Use
const
for values that do not change (preferred in most cases).const apiUrl = "https://api.example.com";
-
Use
let
for variables that will change.let counter = 0; counter += 1;
-
Avoid
var
in modern JavaScript, as it can lead to bugs due to its function-scoped behavior.
Q8: What’s the performance difference between let
, const
, and var
?
In modern JavaScript engines, there’s minimal performance difference between let
, const
, and var
. The choice primarily impacts code readability and maintainability, not execution speed.
Summary Table
Feature | var |
let |
const |
Scope | Function | Block | Block |
Redeclaration | Allowed | Not Allowed | Not Allowed |
Hoisting | Yes (initialized undefined ) |
Yes (TDZ applies) | Yes (TDZ applies) |
Reassignment | Allowed | Allowed | Not Allowed |
Global Object Property | Yes | No | No |
Final Thoughts
When working with JavaScript:
-
Prefer
const
whenever possible for variables that won’t change. -
Use
let
for variables that will change during the program's execution. -
Avoid using
var
unless you're maintaining older codebases.
Mastering let
, const
, and var
will help you write cleaner, safer, and more predictable JavaScript code! 🚀